Comparing for Loop with ES6 forEach Method
JavaScript is a client-side scripting language (forget Node.js). When writing code, we should be careful because our code is supposed to run on all devices rather than just gaming laptops or MacBooks. We should not give much load to the browser.
ES6 a.k.a ES2015 introduced a lot of features especially the array functions
forEach
, map
, filter
, etc. Many people believe forEach is an alternative for the for loop. Is that true? Let’s check some facts.
Before starting, let’s check what is for loop and what is forEach.
For Loop
For is a keyword, to create looping statements, just like while and do…while. JS engine can directly understand what it is.
forEach
forEach is a function defined in
Array.prototype
, just like all other functions. The engine can’t understand it directly. It needs to get the function definition in order to execute it.For vs forEach
Choosing one over another will have huge impact on performance. When you have to deal with an array of bigger size, choosing the right one matters.
Let’s see an example below.
The above code will log 1–100K on the console along with the time it takes to execute the function. The result is given below (on a laptop with 6Gb Ram)
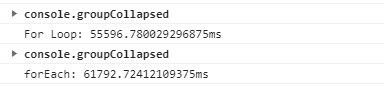
forEach
took 6 more seconds to execute it. logging on console usually takes some time. So 50+ seconds are reasonable. Let’s see another example.The above will simply copy an array of 100K entries to 2 other arrays. Instead of
a=arr
and b=arr
, we push the entries one by one. The time taken is given below.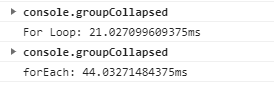
forEach took more than twice the time taken by for loop.
When you are dealing with a small array, you can choose forEach as it is much simpler to write and they don’t have much difference in performance.
Why do people fall for forEach?
- forEach is easy to write. Call back functions are used.
- No index variable is required. callback functions can directly access values one by one.
- Little cleaner than for loop.
Things they forget when moving to forEach
- Performance. for loop is a native way by the engine whereas forEach is provided by Array object.
- There’s no way to break a forEach. If you want to stop a forEach function, you need to throw an exception. And that’s not what exceptions are intended for.
If you are dealing with small arrays, you can use
forEach
method but if you’re dealing with arrays of bigger size, you should be careful.
Comments
Post a Comment